Function tags
Function tags provide many functions we can use to perform common operations, most of which are for String manipulation, such as String COncatenation, Split String, etc.
Here are some tags that you can refer to before looking at examples.
Tag | Description |
fn:contains() | It is used to test if an input string containing the specified substring in a program. |
fn:containsIgnoreCase() | It is used to test if an input string contains the specified substring as a case insensitive way. |
fn:endsWith() | It tests whether an input string ends with the specified suffix. |
fn:escapeXml() | It escapes the characters that would be interpreted as XML markup. |
fn:indexOf() | It returns an index within a string of the first occurrence of a specified substring. |
fn:trim() | It removes the blank spaces from both ends of a string. |
fn:startsWith() | It is used to check whether the given string starts with a particular string value. |
fn:split() | It splits the string into an array of substrings. |
fn:toLowerCase() | It converts all the characters of a string to lowercase. |
fn:toUpperCase() | It converts all the characters of a string to upper case. |
fn:substring() | It returns the subset of a string according to the given start and end position. |
fn:substringAfter() | It returns the subset of the string after a specific substring. |
fn:substringBefore() | It returns the subset of the string before a specific substring. |
fn:length() | It returns the number of characters inside a string or items in a collection. |
fn:replace() | It replaces all the occurrences of a string with another string sequence. |
Example
<%@ page contentType = "text/html; charset=utf-8" %>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<%@ taglib prefix="fn" uri="http://java.sun.com/jsp/jstl/functions" %>
<style>
@import url('https://fonts.googleapis.com/css2?family=Josefin+Sans&display=swap');
</style>
<html>
<head><title>Function Tag</title></head>
<body style="font-family: 'Josefin Sans', sans-serif;">
<c:set var="str1" value="Happy days!"/>
<c:set var="str2" value="app" />
<c:set var="tokens" value="1,2,3,4,5,6,7,8,9,10" />
length(str1) = ${fn:length(str1)} <br>
toUpperCase(str1) = "${fn:toUpperCase(str1)}" <br>
toLowerCase(str1) = "${fn:toLowerCase(str1)}" <br>
substring(str1, 3, 6) = "${fn:substring(str1, 3, 6)}" <br>
substringAfter(str1, str2) = "${fn:substringAfter(str1, str2)}" <br>
substringBefore(str1, str2) = "${fn:substringBefore(str1, str2)}" <br>
trim(str1) = "${fn:trim(str1)}" <br>
replace(str1, src, dest) = "${fn:replace(str1, " ", "-")}" <br>
indexOf(str1, str2) = "${fn:indexOf(str1, str2)}" <br>
startsWith(str1, str2) = "${fn:startsWith(str1, 'Fun')}" <br>
endsWith(str1, str2) = "${fn:endsWith("Using", str1)}" <br>
contains(str1, str2) = "${fn:contains(str1, str2)}" <br>
containsIgnoreCase(str1, str2) = "${fn:containsIgnoreCase(str1, str2)}" <br>
<c:set var="array" value="${fn:split(tokens, ',')}" />
join(array, "-") = "${fn:join(array, "-")}" <br>
escapeXml(str1) = "${fn:escapeXml(str1)}" <br>
</body>
</html>
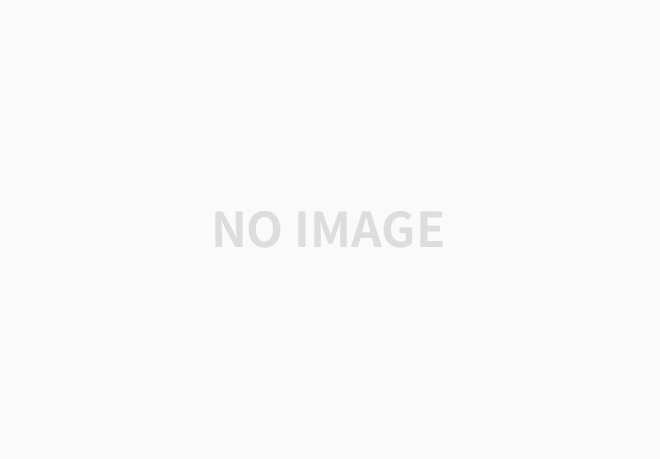
SQL tags
SQL tags support interaction with relational databases such as Oracle, MySql etc. Using JSTL SQL tags, we can run database queries. We first need to create a table with the totoro account to demonstrate.
create table test(
num number,
name varchar2(10),
primary key(num) );
In the code, first, we insert the data and print out the data with the select SQL.
<%@ page language="java" contentType="text/html; charset=EUC-KR"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core"%>
<%@ taglib prefix="sql" uri="http://java.sun.com/jsp/jstl/sql"%>
<html>
<head>
<title>JSTL sql Tags</title>
</head>
<body>
<sql:setDataSource var="conn" driver="oracle.jdbc.driver.OracleDriver"
url="jdbc:oracle:thin:@localhost:1521:xe" user="totoro"
password="totoro123" />
<sql:update dataSource="${conn}">
INSERT INTO test (num, name) VALUES (1, 'Meadow')
</sql:update>
<sql:update dataSource="${conn}">
INSERT INTO test (num, name) VALUES (2, 'Dodo')
</sql:update>
<sql:update dataSource="${conn}">
INSERT INTO test (num, name) VALUES (3, 'Forest')
</sql:update>
<sql:update dataSource="${conn}">
INSERT INTO test (num, name) VALUES (4, 'Jenny')
</sql:update>
<sql:query var="rs" dataSource="${conn}">
SELECT * FROM test WHERE name=?
<sql:param>Meadow</sql:param>
</sql:query>
<c:forEach var="data" items="${rs.rows}">
<c:out value="${data['num']}" />
<c:out value="${data['name']}" />
<br>
</c:forEach>
</body>
</html>
Search the table with select * from test; , you will see the inserted data.
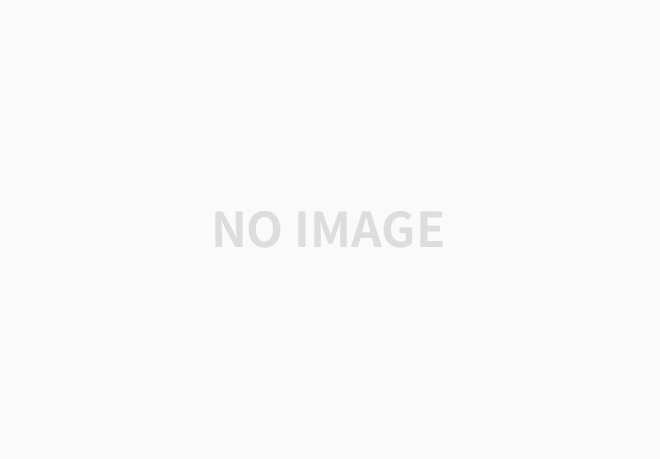
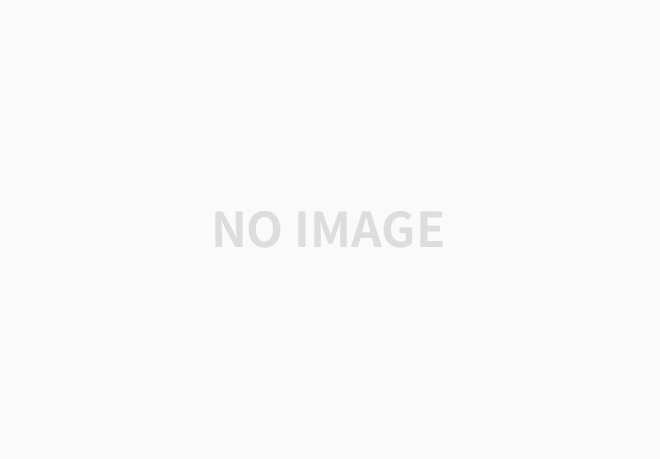
'Java > JSP' 카테고리의 다른 글
JSP) JSTL - Internationalization tags (0) | 2022.09.19 |
---|---|
JSP) JSTL (JSP Standard Tag Library) - Basic / Core tags (0) | 2022.09.17 |
JSP) EL(Expression Language) (0) | 2022.09.16 |
JSP) Model2 - Java Servlet 1 (0) | 2022.09.15 |
JSP) Model1 vs Model2 (0) | 2022.09.15 |