To develop with the Model2 MVC patterns, we need to know Java Servlet, EL(Expression Language), and JSTL(JSP Standard Tag Library), and we already covered the Java Servlet class in the last posts. If you want to go check out, please click the link below.
2022.09.15 - [JSP] - JSP) Model2 - Java Servlet 1
2022.09.16 - [Codes & Projects] - JSP) Model2 - Java Servlet 2
In this post, we will discuss Expression Language, which we use instead of Expression tags in the model2, we use Expression language. It looks different from the expression tags we have been using, but once we get used to it, it will be easier and simpler.
For example, param is used as${param.name} and sessionScope is used as${sessionScope.id}
For a better understanding, let us look through the examples.
Example 1
Arithmetic Operators
To print out the EL on the browser, you must use "\".
<TR>
<TD>\${2 + 5}</TD>
<TD>${2 + 5}</TD>
</TR>
To divide, you can use either "/" or "div" and the result will be the same. "mod" is to get the remainder.
<TR>
<TD>\${4/5}</TD>
<TD>${4/5}</TD>
</TR>
<TR>
<TD>\${5 div 6}</TD>
<TD>${5 div 6}</TD>
</TR>
<TR>
<TD>\${5 mod 7}</TD>
<TD>${5 mod 7}</TD>
</TR>
Comparison Operators
"<" is same as "gt"(greater) and ">" is same as "le"(less).
<TR>
<TD>\${2 < 3}</TD>
<TD>${2 < 3}</TD>
</TR>
<TR>
<TD>\${2 gt 3}</TD>
<TD>${2 gt 3}</TD>
</TR>
<TR>
<TD>\${3.1 le 3.2}</TD>
<TD>${3.1 le 3.2}</TD>
</TR>
Conditional Operators
<TR>
<TD>\${(5 > 3) ? 5 : 3}</TD>
<TD>${(5 > 3) ? 5 : 3}</TD>
</TR>
Implicit Objects
<TR>
<TD>\${header["host"]}</TD>
<TD>${header["host"]}</TD>
</TR>
<TR>
<TD>\${header["user-agent"]}</TD>
<TD>${header["user-agent"]}</TD>
</TR>
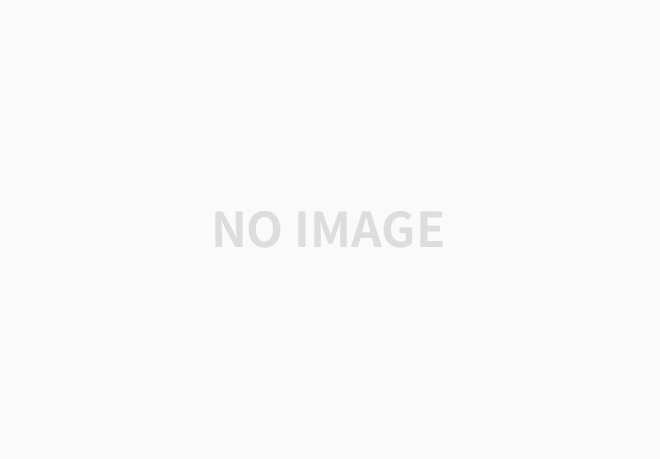
Example 2
Processing parameter value
<%@ page contentType="text/html;charset=utf-8"%>
<% request.setCharacterEncoding("utf-8");%>
<HTML>
<HEAD>
<TITLE>EL Example2</TITLE>
</HEAD>
<BODY>
<H3>Processing parameter value</H3>
<P>
<FORM action="eLEx2.jsp" method="post">
Name : <input type="text" name="name" value="${param['name']}">
<input type="submit" value="Submit">
</FORM>
<P>
Hello <%=request.getParameter("name") %>, how are you today? <br>
Would you like to have a cup of tea, ${param.name}?
</BODY>
</HTML>
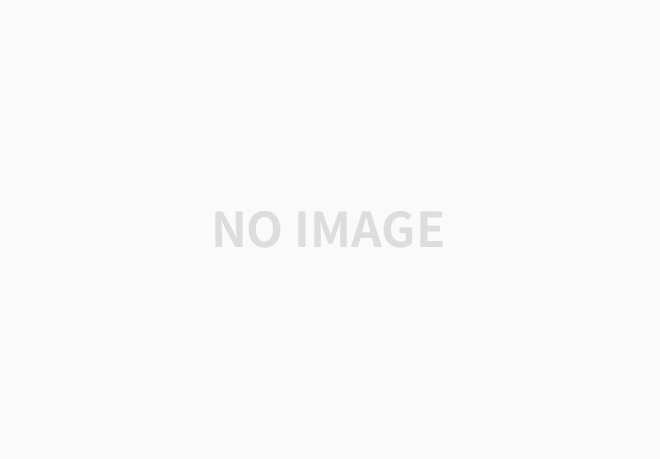
The followings bring the same result, but as you can see, EL is simpler than JSP expression tag.
You can also use ${param['name']}.
Hello <%=request.getParameter("name") %>, how are you today? <br>
Would you like to have a cup of tea, ${param.name}?
${param['name']}
There are several ELs that you can use simpler way.
Object | EL | JSP expression tag |
param | ${param.name} | <%=request.getParameter("name"%> |
sessionScope | ${sessionScope.id} | <%=session.getAttribute("id")%> |
requestScope | ${requestScope.page} | <%=request.getAttribute("page")%> |
Example 3
Product.java
package jspbook;
public class Product {
// Products (Array)
private String[] productList = {"item1","item2","item3","item4","item5"};
// Variables
private int num1 = 10;
private int num2 = 20;
public int getNum1() {
return num1;
}
public int getNum2() {
return num2;
}
public String[] getProductList() {
return productList;
}
}
productList.jsp
<%@ page language="java" contentType="text/html; charset=utf-8"
%>
<html>
<head>
<meta charset="utf-8">
<title>EL Example 3</title>
</head>
<body>
<center>
<H2>Product list</H2>
<HR>
<form method="post" action="productSel.jsp">
<jsp:useBean id="product" class="jspbook.Product" scope="session"/>
<select name="sel">
<%
for(String item : product.getProductList()) {
out.println("<option>"+item+"</option>");
}
%>
</select>
<input type="submit" value="Select"/>
</form>
</center>
</body>
</html>
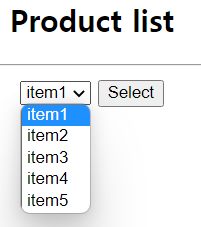
productSel.jsp
<%@page import="jspbook.Product"%>
<%@ page language="java" contentType="text/html; charset=utf-8"%>
<html>
<head>
<meta charset="utf-8">
<title>EL Example 3</title>
</head>
<body>
<center>
<H2>Selected product</H2>
<HR>
1. (EL) You selected : ${param.sel} <br>
1. (Expression tag) You selected : <%=request.getParameter("sel") %><br>
2. num1 + num2 = ${product.num1+product.num2} <br>
<%
Product pro = (Product) session.getAttribute("product");
%>
2.(Expression tag) num1 + num2 = <%=pro.getNum1() + pro.getNum2()%>
</center>
</body>
</html>
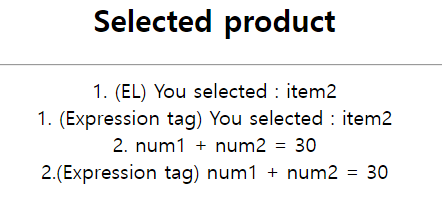
As you explored in this post, Expression Language is often simpler, so as we learn, we will use it more often than the expression tags of the JSP.
'Java > JSP' 카테고리의 다른 글
JSP) JSTL - Internationalization tags (0) | 2022.09.19 |
---|---|
JSP) JSTL (JSP Standard Tag Library) - Basic / Core tags (0) | 2022.09.17 |
JSP) Model2 - Java Servlet 1 (0) | 2022.09.15 |
JSP) Model1 vs Model2 (0) | 2022.09.15 |
JSP) JSP and Oracle (0) | 2022.08.31 |