Starting a new Spring Framework Project is similar to creating a Dynamic Web Project in Eclipse. However, the structure is a bit more complicated if it is your very first time.
So in this post, we will discuss creating a spring project from scratch.
Create a spring project on STS and a new account on Oracle.
After then, create the folders and files.
Configuration file settings in order
1) pom.xml
2) web.xml
3) servlet-context.xml
4) configuration.xml
5) board.xml
6) root-context.xml
1. Create a new account
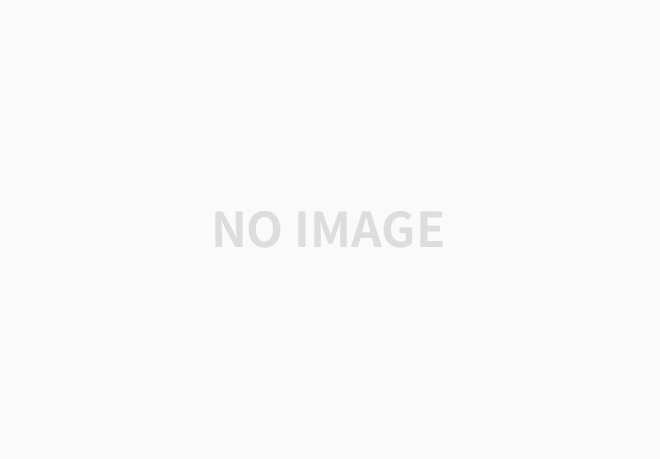
2. Create a Spring Legacy Project.
Fill out the project name, and select the Spring MVC Project.
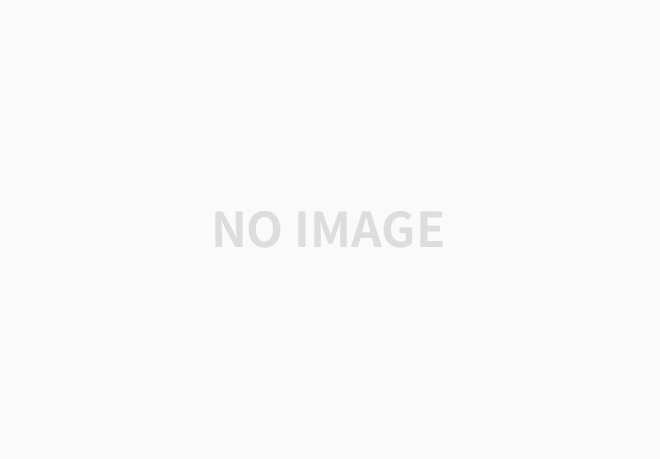
Write the top-level package in the reverse order of the normal website URLs.
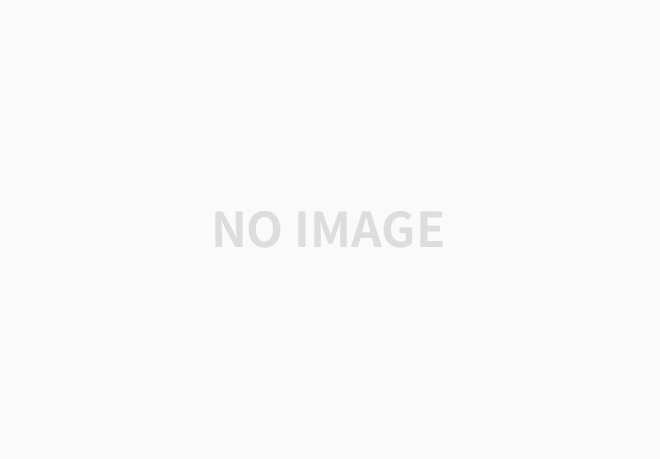
Once it is created, you will see this.
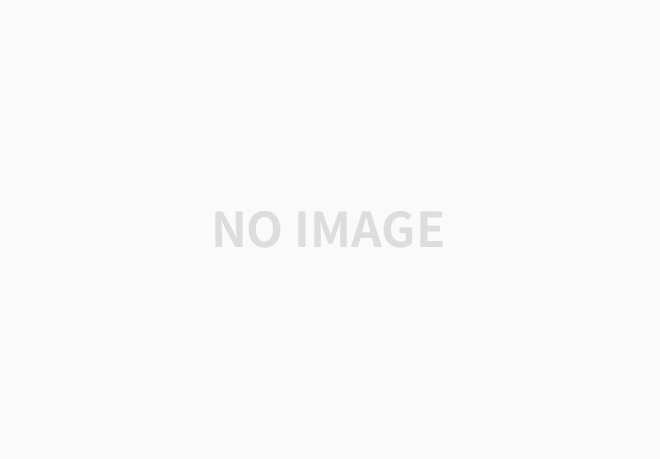
To see if it works, run it on the server.
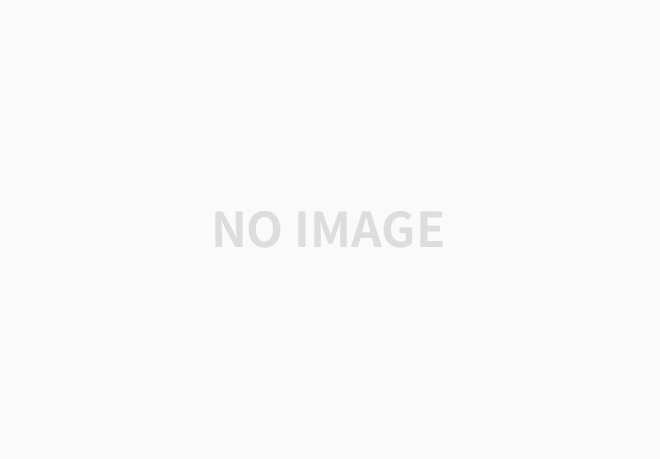
You will see this on the Server.
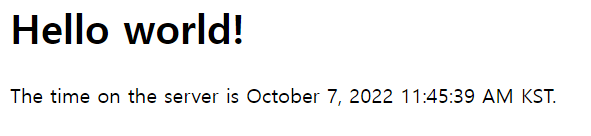
3. Create sql folder and sql file. Connect to the new account.
4. Create a new table and sequence.
-- Bulletin board
select * from tab;
select * from seq;
select * from myboard;
create table myboard(
no number primary key,
writer varchar2(20),
passwd varchar2(20),
subject varchar2(50),
content varchar2(100),
readcount number,
register date );
create sequence myboard_seq;
5. Set pom.xml and run on the server to see if it is set well.
6. Set web.xml. Include encoding tags.
7. Set HomeController.java.
8. Create an index.jsp in webapp folder. Link to the "test.do".
You must keep testing the project every time you create a new file.
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
</head>
<body>
<script>
location.href="test.do";
</script>
</body>
</html>
9. Set servlet-context.xml. Set the base package as myspring and create a new myspring folder containing controller, dao, model, and service folders.
10. Make BoardController.java,BoardService.java, and BoardDao.java in each folder.
11. In the BoardController class, add @Controller, @Autowired annotations. Create the same annotations with other classes as below.
BoardService - @Service, @Autowired
BoardDao - @Repository, @Autowired
Please see the picture below to understand what to put in the classes.
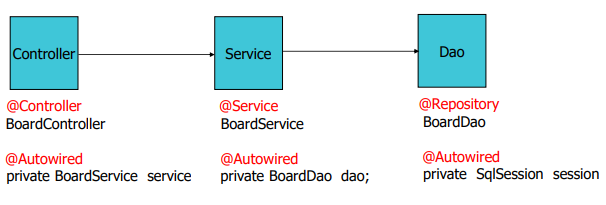
12. Create Board.java in the model folder.
package myspring.model;
import java.util.Date;
public class Board {
private int no;
private String writer;
private String passwd;
private String subject;
private String content;
private int readcount;
private Date register;
public int getNo() {
return no;
}
public void setNo(int no) {
this.no = no;
}
public String getWriter() {
return writer;
}
public void setWriter(String writer) {
this.writer = writer;
}
public String getPasswd() {
return passwd;
}
public void setPasswd(String passwd) {
this.passwd = passwd;
}
public String getSubject() {
return subject;
}
public void setSubject(String subject) {
this.subject = subject;
}
public String getContent() {
return content;
}
public void setContent(String content) {
this.content = content;
}
public int getReadcount() {
return readcount;
}
public void setReadcount(int readcount) {
this.readcount = readcount;
}
public Date getRegister() {
return register;
}
public void setRegister(Date register) {
this.register = register;
}
}
13. Create a board folder in the views folder. In the board folder, create the first file, boardform.jsp.
14. Change the link in the index file to the "boardform.do".
15. In the controller class, connect the boardform.do with the @RequestMapping annotation.
// Boardform
@RequestMapping("boardform.do")
public String boardform() {
return "board/boardform";
}
16. Set configuration.xml.
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE configuration PUBLIC "-//mybatis.org//DTD Config 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-config.dtd">
<configuration>
<typeAliases>
<typeAlias alias="board" type="myspring.model.Board" />
</typeAliases>
</configuration>
17. Create a mapper file, board.xml.
18. Set root-context.xml to connect to the database.
You need to keep this order of configuration files to make the project run.
19. To insert the contents in the database, go to the controller class and add the codes to connect DAO Class.
BoardController.java
// Write a new post
@RequestMapping("boardwrite.do")
public String boardwrite(Board board, Model model) {
int result = bs.insert(board);
if(result == 1) System.out.println("Successfully posted.");
model.addAttribute("result", result);
return "board/insertresult";
}
20. In the service class and DAO Class, add the insert() method.
BoardService.java
public int insert(Board board) {
return dao.insert(board);
}
BoardDao.java
public int insert(Board board) {
return session.insert("insert", board);
}
21. In the board.xml, add insert SQL. The parameter type, the board is the alias set in the configuration file.
Do not put a semicolon after the SQL.
<!-- New post -->
<insert id="insert" parameterType="board"> <!-- board: Alias of Configuration.xml -->
insert into myboard values(myboard_seq.nextval,#{writer},
#{passwd}, #{subject}, #{content},0, sysdate)
</insert>
22. Create a new file, insertresult.jsp.
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<c:if test="${result == 1 }">
<script>
alert("Successfully posted.");
location.href="boardlist.do";
</script>
</c:if>
<c:if test="${result != 1 }">
<script>
alert("Failed to post");
history.go(-1);
</script>
</c:if>
23. Add codes in the controller class to see the post list. Also, include the codes for pagination.
// Post list & pagination
@RequestMapping("boardlist.do")
public String boardlist(HttpServletRequest request, Model model) {
int page = 1; // current page no.
int limit = 10; // limit posts on the page
if(request.getParameter("page") != null) {
page = Integer.parseInt(request.getParameter("page"));
}
int startRow = (page - 1) * limit + 1;
int endRow = page * limit;
int listcount = bs.getCount(); // Total data count
System.out.println("listcount: " + listcount);
List<Board> boardlist = bs.getBoardList(page); // Post List
System.out.println("boardlist:" + boardlist);
24. In the service class and the DAO class, add the getCount() method.
BoardService.java
public int getCount() {
return dao.getCount();
}
BoardDao.java
public int getCount() {
return session.selectOne("count");
}
25. Add select SQL in the board.xml.
26. Edit the index.jsp.
location.href="boardlist.do";
27. In the BoardController.java, add the code showing the post list.
28. In the Service class and the DAO class, ass the List part.
BoardService.java
public List<Board> getBoardList(int page) {
return dao.getBoardList(page);
}
BoardDao.java
public List<Board> getBoardList(int page) {
return session.selectList("list", page);
}
29. Add the list id in the board.xml.
You can use > / < means "greater than"/ "less than" in xml files which has the same role as the <![CDATA[]]>.
<!-- Post list -->
<select id="list" parameterType="int" resultType="board">
<![CDATA[
select * from (select rownum rnum, board.* from (
select * from myboard order by no desc) board )
where rnum >= ((#{page}-1) * 10 + 1) and rnum <= (#{page} * 10)
]]>
</select>
30. Add the codes in the Controller class for the start and end pages.
// Total page
int pageCount = listcount / limit + ((listcount%limit == 0)? 0 : 1);
int startPage = ((page - 1) / 10) * limit + 1; //1, 11, 21,..
int endPage = startPage + 10 - 1; //10, 20, 30..
if(endPage > pageCount) endPage = pageCount;
model.addAttribute("page", page);
model.addAttribute("listcount", listcount);
model.addAttribute("boardlist", boardlist);
model.addAttribute("startPage", startPage);
model.addAttribute("endPage", endPage);
return "board/boardlist";
}
31. To demonstrate, insert the data on Oracle.
insert into myboard values(myboard_seq.nextval, 'Meadow', '111', 'Spring & MyBatis', 'Bulletin Board Project',0,sysdate);
Continue in the next post.
'Spring & Spring Boot' 카테고리의 다른 글
Spring Boot) Basics of Spring Boot /Lombok (0) | 2022.10.18 |
---|---|
Spring) How to start a new Spring Framework Project[2] (0) | 2022.10.14 |
Spring) Spring with MyBatis / Configuration (0) | 2022.10.08 |
Spring) Interceptor (0) | 2022.10.06 |
Spring) Basic Structure and Annotations (0) | 2022.10.05 |