What is Spring Boot? Spring Boot is an extension of Spring, which eliminates the boilerplate configurations required for setting up a Spring application.
Features
Spring Boot enables the development of independent running spring applications because Tomcat and Jetty are already embedded. It manages libraries with Maven/Gradle using Integrated Starter and provides automated spring settings which means you don't need six configuration files as Spring.
Also, what makes it more convenient is that it does not require cumbersome XML settings. Spring Boot also provides Spring Actuator which is used for monitoring and managing applications.
If you already have higher than STS3.0, you can easily create a Spring Boot Project.
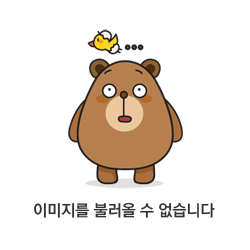
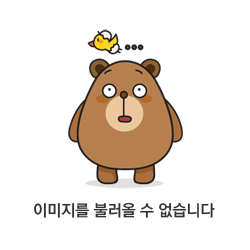
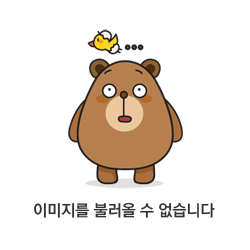
There are many dependencies that you can add easily such as websocket, NoSQL, SQL, MyBatis Framework, and so on. Here, check the Spring web box to add the dependency.
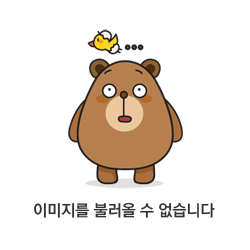
Once we create the project, to check if it is created well, run it on the Spring Boot App.
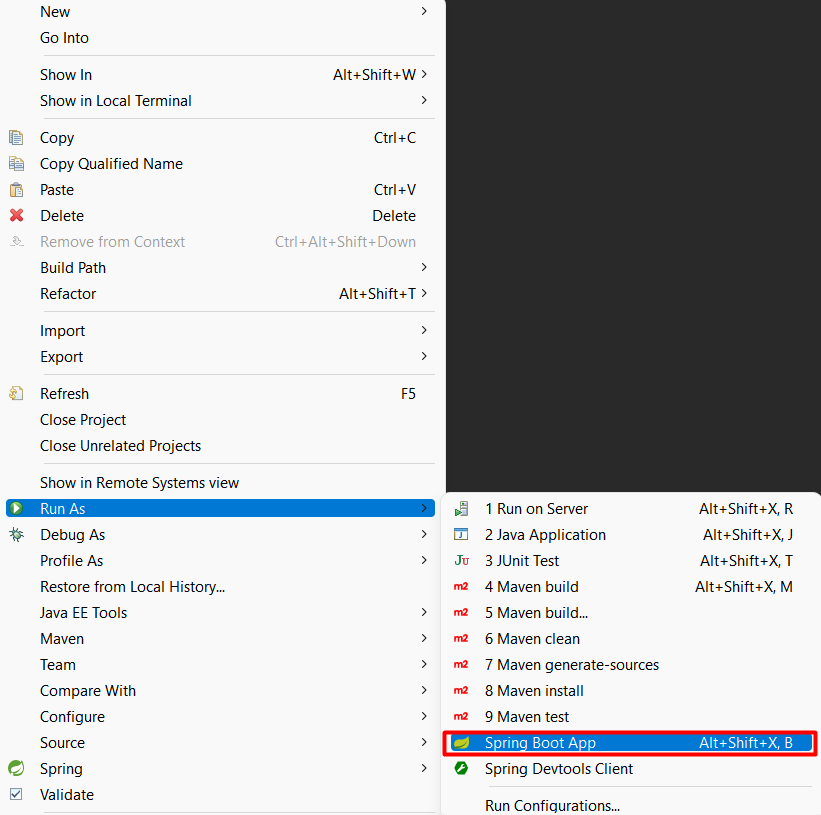
If you see this on the console, it means it is set well!
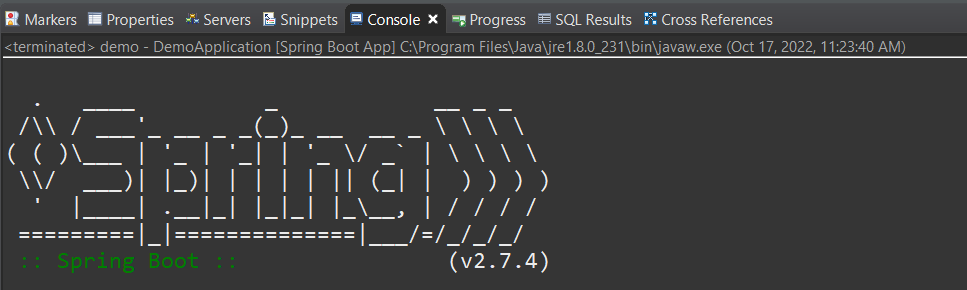
Configuration
pom.xml
configuration.xml
mapper class.
There are no servlet-context.xml, root-context.xml, and web.xml.
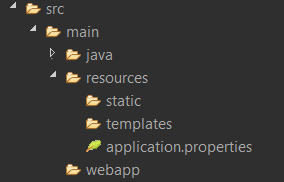
In the static folder, we will save css related files and in the templates folder, we will save HTML files.
In Spring Boot, you need to make controller classes by yourself, unlike Spring. So, create the controller folder in the demo folder, and create SampleController.java.
The application.properties file is empty, you will add the basic configurations that you want to set such as a port number and prefix and suffix. Depending on the functions of the projects, you can add other properties in this file. Other then this, Spring and Spring Boot are very similar.
Lombok
Lombok is something that makes the project even simpler. Once you use Lombok function, you don't need getter and setter methods in DTO class. Instead of these methods, with this Lombok function, you can use simple annotations.
To compare the projects with and without Lombok function, we will create a new project.
Spring Starter Project without Lombok
Create a new project and check the boxes of the dependencies that you need for the project.
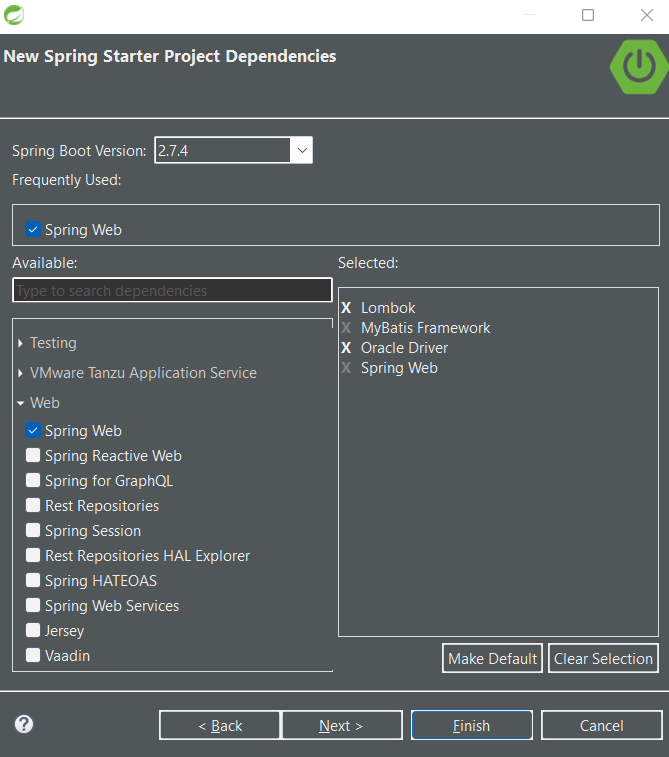
The dependencies that you checked will be downloaded automatically once you click the Finish button and you will see the dependencies in pom.xml.
In application.properties, you won't see anything because, in Spring Boot, we need to add the configurations.
So, we will set a port number and prefix/ suffix here.
In main / java / com / example / demo / model, create a DTO Class, Member.java.
In this project, we are not using the Lombok, so we will create getter and setter methods.
In the same demo folder, create a controller package and create SampleController.java.
Create index.jsp to connect the controller class. In the index.jsp, link "main" and create main.jsp.
In this demonstration, we will make a login form.
main.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Log in</title>
</head>
<body>
<form method="post" action="send">
ID: <input type=text name="id"> <br>
Password: <input type=text name="passwd"> <br>
<input type="submit" value="Log in">
</form>
</body>
</html>
So the flow will be index.jsp -> SampleController.java -> main.jsp.
In terms of encoding, you don't have to care, because Spring boot will automatically take care of it.
Spring Starter Project with Lombok
With the Lombok dependency, you don't have to create getter and setter methods, or other constructors like toString() and Equals(). First, add the dependency in pom.xml and we need to download the dependency from the Lombok website(https://projectlombok.org/all-versions).
To execute the downloaded file, you need to do two things: add dependency and install it in STS.
Since we already added the dependency, we will do the latter one.
Open the command prompt and insert the commands below.

Click the Install/Update button and you will see the alert "Install successful".
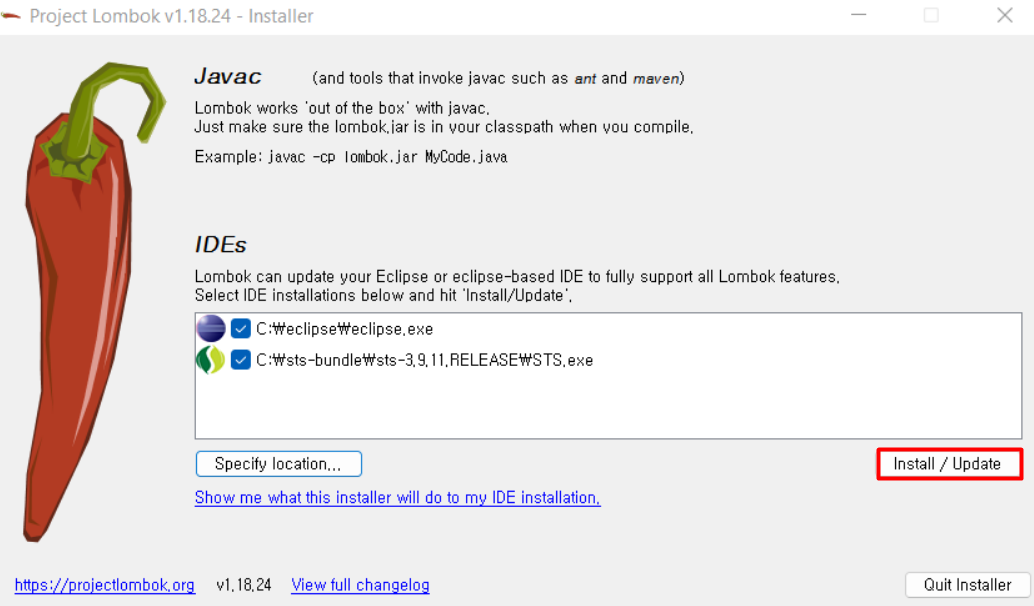
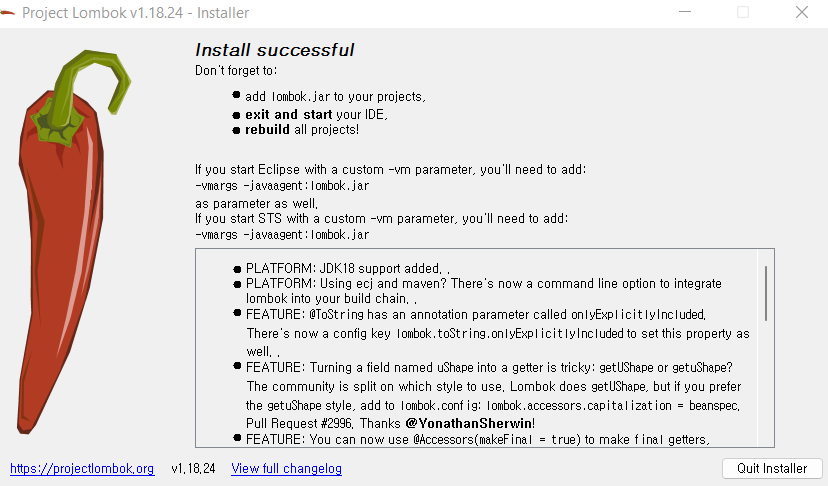
It is all set and now, we can use the Lombok function.
In the Member.java, comment out the getter and setter methods.
Instead of getter and setter methods, we will use annotations.
@Getter
@Setter
// @Data
public class Member {
private String id;
private String passwd;
}
As you can see, if you use the Lombok function, the codes will be still shorter and simpler.
'Spring & Spring Boot' 카테고리의 다른 글
Spring Boot) Spring Boot with MyBatis (0) | 2022.11.19 |
---|---|
Spring Boot) Thymeleaf (0) | 2022.10.19 |
Spring) How to start a new Spring Framework Project[2] (0) | 2022.10.14 |
Spring) How to start a new Spring Framework Project[1] (0) | 2022.10.11 |
Spring) Spring with MyBatis / Configuration (0) | 2022.10.08 |