Str, List and Dictionary are the most used data structure.
To save data in a dictionary, you must save the key and value(data) together, and you will use the key to get the value. Like set, dictionary is not an ordered data structure as well. If there are multiple overlapped key and we search the same key, it will print out the value of the last key.
Example
{ 'key ' : 'value' } = { 'Name' : code }
names = {'Mary':10999, 'Sams':2111, 'Amy':9778, 'Tom':20245 , 'Mike':27115,
'Bob':5887, 'Kelly':7855, 'Mary':100}
print(type(names)) # <class 'dict'>
print(names) # {'Mary': 100, 'Sams': 2111, 'Amy': 9778, 'Tom': 20245, 'Mike': 27115, 'Bob': 5887, 'Kelly': 7855}
print(names['Amy']) # 9778
print(names['Tom']) # 20245
print(names['Mary']) # 100
keys()
names = {'Mary':10999, 'Sams':2111, 'Amy':9778, 'Tom':20245 , 'Mike':27115,
'Bob':5887, 'Kelly':7855}
for k in names.keys():
# print('Key:%s\t Value:%d'%(k, names[k]))
# print('Key:', k, ' Value:', names[k])
print('Key:{0} Value:{1}'.format(k, names[k]))
values()
result = names.values()
print(type(result)) # <class 'dict_values'>
print(result) # dict_values([10999, 2111, 9778, 20245, 27115, 5887, 7855])
list = list(result) # list()
print(type(list)) # <class 'list'>
print(list) # [10999, 2111, 9778, 20245, 27115, 5887, 7855]
count()
count = sum(list)
print('Sum:%d'%count)
items()
for item in names.items():
print(item) # ('Mary', 10999)
# ('Sams', 2111)
# ...
for k, v in names.items():
print(k,':',v) # Mary : 10999
# Sams : 2111
# ...
To edit dictionary values
names = {'Mary':10999, 'Sams':2111, 'Aimy':9778, 'Tom':20245 , 'Michale':27115,
'Bob':5887, 'Kelly':7855}
print(names)
print(names['Aimy']) # 9778
print(names.get('Aimy')) # 9778
names['Aimy'] = 10000
print(names['Aimy']) # 10000
To delete dictionary values
names = {'Mary':10999, 'Sams':2111, 'Aimy':9778, 'Tom':20245 , 'Michale':27115,
'Bob':5887, 'Kelly':7855}
del names['Sams']
del names['Bob']
# To check
print(names)
# Delete all
names.clear()
# To check
print(names) # {}
mydic = {}
Example
names = {'Mary':10999, 'Sams':2111, 'Aimy':9778, 'Tom':20245 , 'Michale':27115,
'Bob':5887, 'Kelly':7855}
k = input('Enter the name.')
if k in names:
print('%s: %d'%(k, names[k]))
else:
print('There is no ''%s''.'%k)
names[k] = 1000;
print(names)
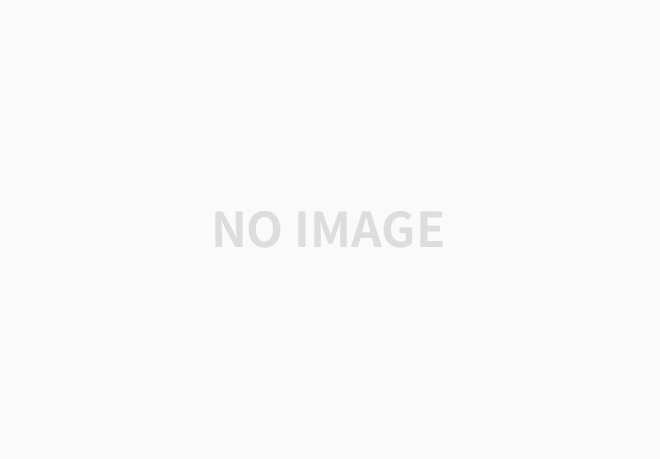
Ascending and descending order of dictionary
names = {'Mary':10999, 'Sams':2111, 'Aimy':9778, 'Tom':20245 , 'Michale':27115,
'Bob':5887, 'Kelly':7855}
def f1(x):
return x[0]
def f2(x):
return x[1]
# 1. key, ascending
result1 = sorted(names)
print('result1:', result1) # ['Aimy', 'Bob', 'Kelly', 'Mary', 'Michale', 'Sams', 'Tom']
result2 = sorted(names.items(), key=f1)
print('result2:', result2) # [('Aimy', 9778), ('Bob', 5887), ('Kelly', 7855), ('Mary', 10999), ('Michale', 27115), 'Tom']
# 2. key, descending
result3 = sorted(names, reverse=True)
print('result3:', result3) # ['Tom', 'Sams', 'Michale', 'Mary', 'Kelly', 'Bob', 'Aimy']
result4 = sorted(names.items(), reverse=True , key=f1)
print('result4:', result4) # [('Tom', 20245), ('Sams', 2111), ('Michale', 27115), ('Mary', 10999), ('Kelly', 7855)y']
# 3. value ascending
result5 = sorted(names.items(), key=f2)
print('result5:', result5) # [('Sams', 2111), ('Bob', 5887), ('Kelly', 7855), ('Aimy', 9778), ('Mary', 10999),
# 4. value descending
result6 = sorted(names.items(), reverse=True , key=f2)
print('result6:', result6) # [('Michale', 27115), ('Tom', 20245), ('Mary', 10999), ('Aimy', 9778), ('Kelly', 7855)
'Python' 카테고리의 다른 글
Python) list (0) | 2022.11.08 |
---|---|
Python) Control statements (0) | 2022.11.06 |
Python) Tuple / Set (0) | 2022.11.03 |
Python) Datatypes - Str (0) | 2022.11.03 |
Python) Variables and Operators (0) | 2022.10.29 |